Introduction to ASP.NET Core: A Modern, Cross-Platform Web Framework
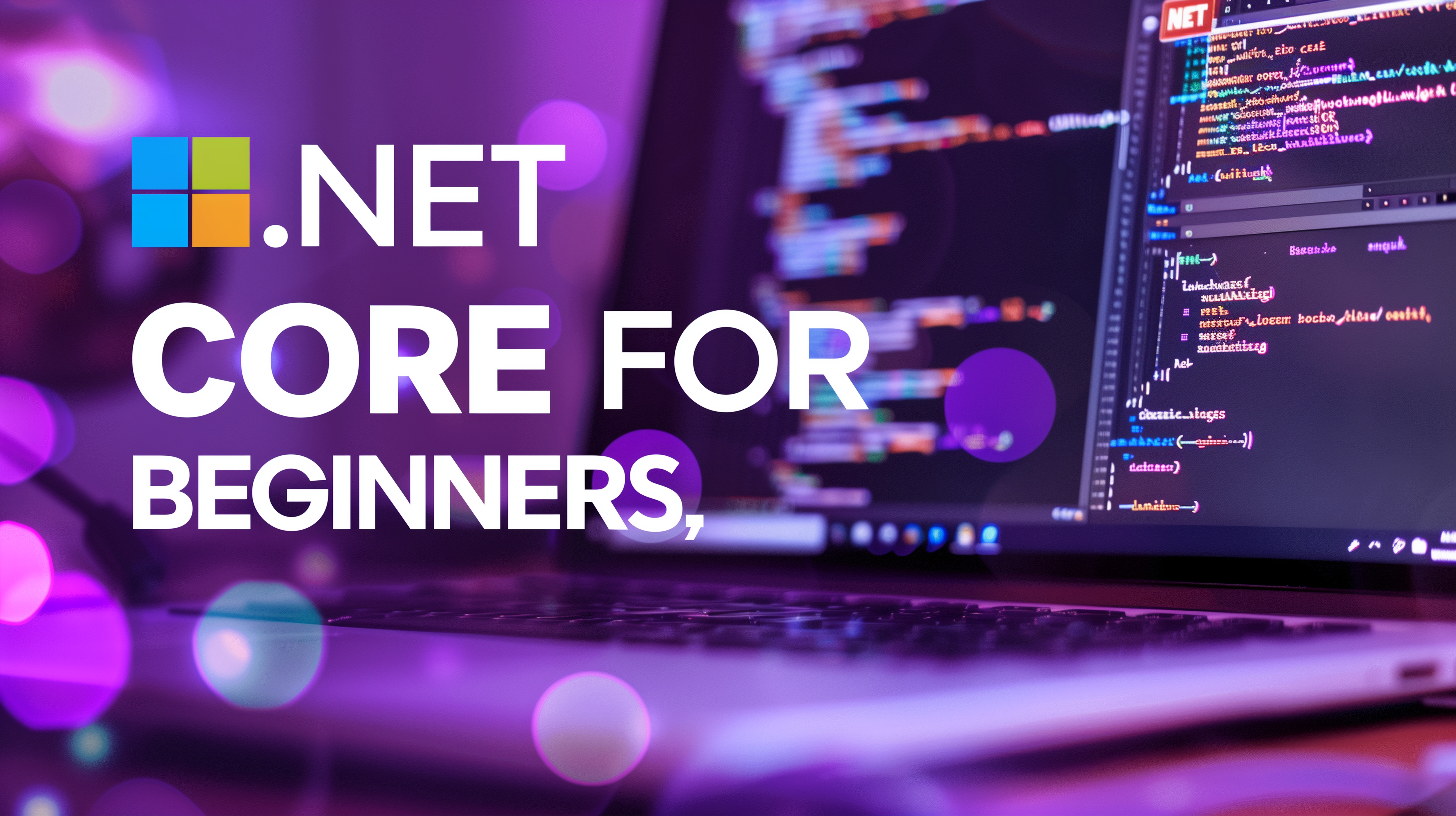
Introduction to ASP.NET Core
ASP.NET Core is a cross-platform, high-performance framework for building modern, cloud-based, Internet-connected applications. It’s a complete rewrite of the original ASP.NET framework, designed to be lightweight, modular, and flexible.
Using ASP.NET Core, developers can build everything from small websites to large enterprise systems, including RESTful APIs and microservices. It is built on the .NET Core runtime, which allows it to run on Windows, macOS, and Linux.
Tip: Refer to the official Microsoft ASP.NET Core documentation for further details, and check out the open-source repository on GitHub.
Cross-Platform
ASP.NET Core applications can run on any operating system that supports .NET Core. This flexibility means you can develop on Windows, macOS, or Linux and then deploy to any of those environments.
Hosting
On the server side, ASP.NET Core applications can be hosted in a variety of environments, including:
- IIS
- Kestrel
- Docker
- Nginx
- Apache
A reverse proxy (e.g., Nginx or Apache) is commonly used to forward incoming requests to Kestrel. This arrangement can help with load balancing, security, SSL termination, and more.
Below is a simple diagram illustrating a typical hosting setup with ASP.NET Core:
Open Source
ASP.NET Core is fully open source, with the code available on GitHub. This means developers from around the world can collaborate on the framework, customize it, and contribute to its evolution.
Cloud-Ready
ASP.NET Core is designed with the cloud in mind. It supports:
- Modern Development Practices (microservices, containerization, CI/CD pipelines)
- Cloud-Native Features (configuration management, logging, health checks)
- Easy Deployment to Azure, AWS, or Google Cloud Platform
Modular
There are four main parts (or “flavors”) of ASP.NET Core:
- ASP.NET Core MVC
- ASP.NET Core Web API
- ASP.NET Core Razor Pages
- ASP.NET Core Blazor
ASP.NET Core MVC
ASP.NET Core MVC is a complete rewrite of the older ASP.NET MVC framework. It follows the Model-View-Controller (MVC) pattern:
MVC provides a way to separate concerns and organize code in a clean, maintainable way. This is the most commonly used approach for building web applications.
ASP.NET Core Web API
If you’re focused solely on controllers and models (without views), ASP.NET Core Web API is your go-to. It’s lightweight, designed for building RESTful APIs, and easily consumed by client applications (e.g., web, mobile, desktop). Your frontend can then be built with any framework you like (e.g., Angular, React, Vue.js).
ASP.NET Core Razor Pages
Razor Pages is a page-based programming model that simplifies building dynamic web pages. It’s layered on top of MVC but is often more straightforward for smaller apps or developers new to ASP.NET Core. Razor Pages let you combine C# and HTML in .cshtml
files, reducing boilerplate.
ASP.NET Core Blazor
Blazor allows developers to write interactive client-side code in C# instead of JavaScript. It comes in two flavors:
- Blazor Server – Runs on the server and communicates with the client via SignalR.
- Blazor WebAssembly – Runs entirely in the browser using WebAssembly.
Focus
In future blog posts, we will dive deeper into ASP.NET Core MVC and ASP.NET Core Web API, since these are the most common scenarios:
- Building robust web applications using MVC
- Building RESTful APIs using Web API
However, keep in mind Razor Pages and Blazor are powerful alternatives that might fit certain project requirements.
Prerequisites
Future posts assume familiarity with:
- C# (OOP concepts like classes, interfaces, inheritance, polymorphism)
- Asynchronous Programming (
async
/await
) - Extension Methods, Lambda Expressions, LINQ
- Basic Web Dev (HTML, CSS, JavaScript, jQuery—at least an intermediate understanding)
If any of these are new or rusty for you, online resources and tutorials (including Microsoft’s official docs) can get you up to speed quickly.
Minimal “Hello World” in ASP.NET Core
To give you a quick taste of ASP.NET Core, here’s a short code snippet for a minimal web app. In a new ASP.NET Core empty project, you can paste this into Program.cs
:
var builder = WebApplication.CreateBuilder(args);
var app = builder.Build();
app.MapGet("/", () => "Hello from ASP.NET Core!");
app.Run();
Conclusion and Next Steps
ASP.NET Core offers:
- Cross-Platform development
- Lightweight & Modular architecture
- Open Source collaboration
- Cloud-Ready deployment options
In the next blog post, we’ll compare ASP.NET Web Forms, ASP.NET MVC, and ASP.NET Core to understand the differences and see why ASP.NET Core is often preferred for modern web development. After that, we’ll get hands-on with creating new ASP.NET Core projects, exploring folder structures, and best practices.
Next Steps
- Make sure you have the .NET 6 or later SDK installed.
- Try out the minimal “Hello World” sample above.
- Familiarize yourself with basic MVC or Web API setups so that when we dive deeper, you’re ready to follow along.
Stay tuned for the comparison post, where we’ll go over the evolution from Web Forms to MVC to ASP.NET Core!
Thanks for reading! If you have any questions or topics you’d like to see covered, feel free to let me know in the comments.
Powered by Froala Editor